The RNA Folding Grammar
The RNA folding grammar as implemented in RNAlib
Specialized Modules:
Introduction
To predict secondary structures composed of the four distinguished loop types introduced before, all algorithms implemented in RNAlib follow a specific recursive decomposition scheme, also known as the RNA folding grammar, or Secondary Structure Folding Recurrences.
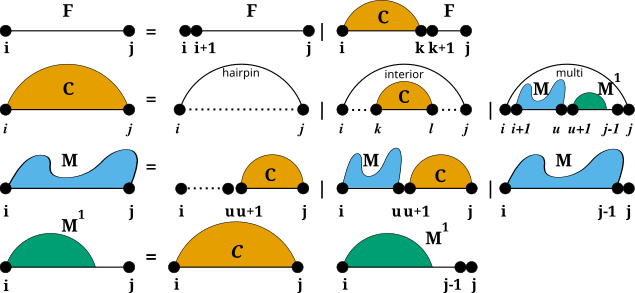
However, compared to other RNA secondary structure prediction libraries, our implementation allows for a fine-grained control of the above recursions through:
Secondary Structure Constraints, that may be applied to both, the individual derivations of the grammar (Hard Constraints) as well as the evaluation of particular loop contributions (Soft Constraints)
Extension of the RNA folding grammar rule set. Here, users may provide additional rules for each of the decomposition stages
F
,C
,M
, andM1
, as well further auxiliary rules.Structured Domains and Unstructured Domains that already implement efficient grammar extensions for self-enclosed structures and unpaired sequence segments, respectively.
Additional Structural Domains
Some applications of RNA secondary structure prediction require an extension of the regular RNA folding grammar. For instance one would like to include proteins and other ligands binding to unpaired loop regions while competing with conventional base pairing. Another application could be that one may want to include the formation of self-enclosed structural modules, such as G-quadruplexes. For such applications, we provide a pair of additional domains that extend the regular RNA folding grammar, Structured Domains and Unstructured Domains.
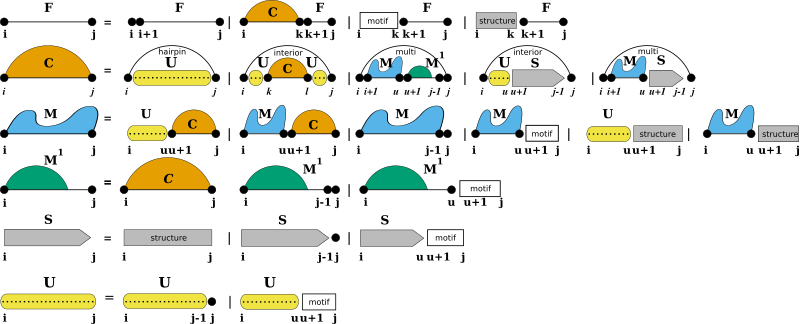
While unstructured domains are usually determined by a more or less precise
sequence motif, e.g. the binding site for a protein, structured domains are
considered self-enclosed modules with a more or less complex pairing pattern.
Our extension with these two domains introduces two production rules to fill
additional dynamic processing matrices S
and U
where we store the
pre-computed contributions of structured domains (S
), and unstructured
domains (U
).
Extending the RNA Folding Grammar
The RNA folding grammar can be easily extended by additional user-provided
rules. For that purpose, we implement a generic callback-based machinery, that
allows one to add rules to the existing decomposition stages F
, C
, M
,
and M1
. If such an extension is not sufficient, users may even extend the
grammar by arbitrary additional derivations.
Additional rules can be supplied for both, MFE and partition function computations. Here, a set of two callback functions must be provided, one for the inside, and another for the outside recursions. The functions will then be called at appropriate steps in the recursions automatically. Each function will be provided with a set of parameters for the decomposition step and a user-defined data pointer that may point to some memory storing any additional data required for the grammar rule.
The detailed API for this mechanism can be found below.
RNA Folding Grammar Extension API
Typedefs
-
typedef struct vrna_gr_aux_s *vrna_gr_aux_t
- #include <ViennaRNA/grammar/basic.h>
A pointer to the auxiliary grammar data structure.
-
typedef char *(*vrna_gr_serialize_bp_f)(vrna_fold_compound_t *fc, vrna_bps_t bp_stack, void *data)
- #include <ViennaRNA/grammar/basic.h>
Function prototype for serializing backtracked base pairs and structure elements into a dot-bracket string.
This function will be called after backtracking in the MFE predictions to convert collected base pairs and other information into a dot-bracket-like structure string.
- Notes on Callback Functions:
This callback allows for changing the way how base pairs (and other types of data) obtained from the default and extended grammar are converted back into a dot-bracket string.
See also
- Param fc:
The fold compound to work on
- Param bp_stack:
The base pair stack
- Param data:
An arbitrary user-provided data pointer
- Return:
A ‘\0’-terminated dot-bracket-like string representing the structure from
bp_stack
(anddata
)
-
typedef int (*vrna_gr_inside_f)(vrna_fold_compound_t *fc, unsigned int i, unsigned int j, void *data)
- #include <ViennaRNA/grammar/mfe.h>
Function prototype for auxiliary grammar rules (inside version, MFE)
This function will be called during the inside recursions of MFE predictions for subsequences from position
i
toj
and is supposed to return an energy contribution in dekacal/mol.- Notes on Callback Functions:
This callback allows for extending the MFE secondary structure decomposition with additional rules.
See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_c(), vrna_gr_add_aux_m(), vrna_gr_add_aux_m1(), vrna_gr_add_aux_aux(), vrna_gr_add_aux_exp_f()
- Param fc:
The fold compound to work on
- Param i:
The 5’ delimiter of the sequence segment
- Param j:
The 3’ delimiter of the sequence segment
- Param data:
An arbitrary user-provided data pointer
- Return:
The free energy computed by the auxiliary grammar rule in dekacal/mol
-
typedef int (*vrna_gr_outside_f)(vrna_fold_compound_t *fc, unsigned int i, unsigned int j, int e, vrna_bps_t bp_stack, vrna_bts_t bt_stack, void *data)
- #include <ViennaRNA/grammar/mfe.h>
Function prototype for auxiliary grammar rules (outside version, MFE)
This function will be called during the backtracking stage of MFE predictions for subsequences from position
i
toj
and is supposed to identify the the structure components that give rise to the corresponding energy contributione
as determined in the inside (forward) step.For that purpose, the function may modify the base pair stack (
bp_stack
) by adding all base pairs identified through the additional rule. In addition, when the rule sub-divides the sequence segment into smaller pieces, these pieces can be put onto the backtracking (bt_stack
) stack. On successful backtrack, the function returns non-zero, and exactly zero (0) when the backtracking failed.- Notes on Callback Functions:
This callback allows for backtracking (sub)structures obtained from extending the MFE secondary structure decomposition with additional rules.
See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_c(), vrna_gr_add_aux_m(), vrna_gr_add_aux_m1(), vrna_gr_add_aux_aux(), vrna_gr_add_aux_exp_f()
- Param fc:
The fold compound to work on
- Param i:
The 5’ delimiter of the sequence segment
- Param j:
The 3’ delimiter of the sequence segment
- Param e:
The energy of the segment [i:j]
- Param bp_stack:
The base pair stack
- Param bt_stack:
The backtracking stack (all segments that need to be further investigated)
- Param data:
An arbitrary user-provided data pointer
- Return:
The free energy computed by the auxiliary grammar rule in dekacal/mol
-
typedef FLT_OR_DBL (*vrna_gr_inside_exp_f)(vrna_fold_compound_t *fc, unsigned int i, unsigned int j, void *data)
- #include <ViennaRNA/grammar/partfunc.h>
Function prototype for auxiliary grammar rules (inside version, partition function)
This function will be called during the inside recursions of partition function predictions for subsequences from position
i
toj
and is supposed to return a Boltzmann factor with energy contribution in cal/mol.- Notes on Callback Functions:
This callback allows for extending the partition function secondary structure decomposition with additional rules.
See also
vrna_gr_add_aux_exp_f(), vrna_gr_add_aux_exp_c(), vrna_gr_add_aux_exp_m(), vrna_gr_add_aux_exp_m1(), vrna_gr_add_aux_exp(), vrna_gr_add_aux_f()
- Param fc:
The fold compound to work on
- Param i:
The 5’ delimiter of the sequence segment
- Param j:
The 3’ delimiter of the sequence segment
- Param data:
An arbitrary user-provided data pointer
- Return:
The partition function omputed by the auxiliary grammar rule with energies in cal/mol
-
typedef FLT_OR_DBL (*vrna_gr_outside_exp_f)(vrna_fold_compound_t *fc, unsigned int i, unsigned int j, void *data)
- #include <ViennaRNA/grammar/partfunc.h>
Functions
-
unsigned int vrna_gr_prepare(vrna_fold_compound_t *fc, unsigned int options)
- #include <ViennaRNA/grammar/basic.h>
Prepare the auxiliary grammar rule data.
Note
This function is mainly for internal use. Users of the auxiliary grammar API usually do not need to call this function except for debugging purposes.
- Parameters:
fc – The fold compound storing the auxiliary grammar rules
options – Options flag(s) that denote what needs to be prepared
- Returns:
non-zero on success, 0 otherwise
-
unsigned int vrna_gr_add_status(vrna_fold_compound_t *fc, vrna_recursion_status_f cb, void *data, vrna_auxdata_prepare_f prepare_cb, vrna_auxdata_free_f free_cb)
- #include <ViennaRNA/grammar/basic.h>
Add status event based data preparation callbacks.
This function binds additional data structures and corresponding callback functions for the auxiliary grammar extension API. This might be helpful whenever certain preparation steps need to be done prior and/or after the actual run of the prediction algorithms.
- Parameters:
fc – The fold compound
cb – The recursion status callback that performs the preparation
data – The data pointer the
cb
callback is working onprepare_cb – A preparation callback function for parameter
data
free_cb – A callback to release memory for
data
- Returns:
The number of status function callbacks bound to the fold compound or 0 on error
-
unsigned int vrna_gr_set_serialize_bp(vrna_fold_compound_t *fc, vrna_gr_serialize_bp_f cb, void *data, vrna_auxdata_prepare_f prepare_cb, vrna_auxdata_free_f free_cb)
- #include <ViennaRNA/grammar/basic.h>
Set base pair stack to dot-bracket string serialization function.
After backtracking secondary structures, e.g. in MFE predictions, the outside algorithm usually collects a set of base pairs that then need to be converted into a dot-bracket string. By default, this conversion is done using the vrna_db_from_bps() function. However, this function only considers nested base pairs and no other type of secondary structure elements.
When extending the recursions by additional rules, the default conversion may not suffice, e.g. because the grammar extension adds 2.5D modules or pseudoknots. In such cases the user should implement its own dot-bracket string conversion strategy that may use additional symbols.
This function binds a user-implemented conversion function that must return a ‘\0’ terminated dot-bracket-like string the same length as the input sequence. The conversion function will then be used instead of the default one. In addition to the base pair stack vrna_bps_t, the user-defined conversion function may keep track of whatever information is neccessary to properly convert the backtracked structure into a dot-bracket string. For that purpose, the
data
pointer can be used, e.g. it can point to the same data as used in any of the grammar extension rules. Theprepare_cb
andfree_cb
callbacks can again be used to control preparation and release of the memorydata
points to. Theprepare_cb
will be called after all the preparations for the grammar extensions and prior the actual inside-recursions. The conversion function callbackcb
will be called after backtracking.See also
- Parameters:
fc – The fold compound
cb – A pointer to the conversion callback function
data – A pointer to arbitrary data that will be passed through to
cb
(may be NULL)prepare_cb – A function pointer to prepare
data
(may be NULL)free_cb – A function pointer to release memory occupied by
data
(may be NULL)
-
void vrna_gr_reset(vrna_fold_compound_t *fc)
- #include <ViennaRNA/grammar/basic.h>
Remove all auxiliary grammar rules.
This function re-sets the fold compound to the default rules by removing all auxiliary grammar rules
- Parameters:
fc – The fold compound
-
unsigned int vrna_gr_add_aux_f(vrna_fold_compound_t *fc, vrna_gr_inside_f cb, vrna_gr_outside_f cb_bt, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/mfe.h>
Add an auxiliary grammar rule for the F-decomposition (MFE version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the F-decomposition, i.e. the external loop decomposition stage.
While the inside rule (
cb
) computes a minimum free energy contribution for any subsequence the outside rule (cb_bt
) is used for backtracking the corresponding structure.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.See also
vrna_gr_add_aux_c(), vrna_gr_add_aux_m(), vrna_gr_add_aux_m1(), vrna_gr_add_aux(), vrna_gr_add_aux_exp_f(), vrna_gr_inside_f, vrna_gr_outside_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_bt – The auxiliary grammar callback for the outside (backtracking) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the MFE F-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_c(vrna_fold_compound_t *fc, vrna_gr_inside_f cb, vrna_gr_outside_f cb_bt, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/mfe.h>
Add an auxiliary grammar rule for the C-decomposition (MFE version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the C-decomposition, i.e. the base pair decomposition stage.
While the inside rule (
cb
) computes a minimum free energy contribution for any subsequence the outside rule (cb_bt
) is used for backtracking the corresponding structure.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_m(), vrna_gr_add_aux_m1(), vrna_gr_add_aux(), vrna_gr_add_aux_exp_c(), vrna_gr_inside_f, vrna_gr_outside_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_bt – The auxiliary grammar callback for the outside (backtracking) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the MFE C-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_m(vrna_fold_compound_t *fc, vrna_gr_inside_f cb, vrna_gr_outside_f cb_bt, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/mfe.h>
Add an auxiliary grammar rule for the M-decomposition (MFE version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the M-decomposition, i.e. the multibranch loop decomposition stage.
While the inside rule (
cb
) computes a minimum free energy contribution for any subsequence the outside rule (cb_bt
) is used for backtracking the corresponding structure.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_c(), vrna_gr_add_aux_m1(), vrna_gr_add_aux(), vrna_gr_add_aux_exp_m(), vrna_gr_inside_f, vrna_gr_outside_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_bt – The auxiliary grammar callback for the outside (backtracking) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the MFE M-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_m1(vrna_fold_compound_t *fc, vrna_gr_inside_f cb, vrna_gr_outside_f cb_bt, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/mfe.h>
Add an auxiliary grammar rule for the M1-decomposition (MFE version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the M1-decomposition, i.e. the multibranch loop components with exactly one branch decomposition stage.
While the inside rule (
cb
) computes a minimum free energy contribution for any subsequence the outside rule (cb_bt
) is used for backtracking the corresponding structure.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_c(), vrna_gr_add_aux_m(), vrna_gr_add_aux(), vrna_gr_add_aux_exp_m1(), vrna_gr_inside_f, vrna_gr_outside_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_bt – The auxiliary grammar callback for the outside (backtracking) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the MFE M1-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_m2(vrna_fold_compound_t *fc, vrna_gr_inside_f cb, vrna_gr_outside_f cb_bt, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/mfe.h>
Add an auxiliary grammar rule for the M2-decomposition (MFE version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the M2-decomposition, i.e. the multibranch loop components with at least two branches.
While the inside rule (
cb
) computes a minimum free energy contribution for any subsequence the outside rule (cb_bt
) is used for backtracking the corresponding structure.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_c(), vrna_gr_add_aux_m(), vrna_gr_add_aux(), vrna_gr_add_aux_exp_m1(), vrna_gr_inside_f, vrna_gr_outside_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_bt – The auxiliary grammar callback for the outside (backtracking) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the MFE M2-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux(vrna_fold_compound_t *fc, vrna_gr_inside_f cb, vrna_gr_outside_f cb_bt, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/mfe.h>
Add an auxiliary grammar rule (MFE version)
This function binds callback functions for auxiliary grammar rules (inside and outside) as additional, independent decomposition steps.
While the inside rule (
cb
) computes a minimum free energy contribution for any subsequence the outside rule (cb_bt
) is used for backtracking the corresponding structure.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.See also
vrna_gr_add_aux_f(), vrna_gr_add_aux_c(), vrna_gr_add_aux_m(), vrna_gr_add_aux_m1(), vrna_gr_add_aux_exp(), vrna_gr_inside_f, vrna_gr_outside_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_bt – The auxiliary grammar callback for the outside (backtracking) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for MFE predictions, or 0 on error.
-
unsigned int vrna_gr_add_aux_exp_f(vrna_fold_compound_t *fc, vrna_gr_inside_exp_f cb, vrna_gr_outside_exp_f cb_out, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/partfunc.h>
Add an auxiliary grammar rule for the F-decomposition (partition function version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the F-decomposition, i.e. the external loop decomposition stage.
While the inside rule (
cb
) computes the partition function for any subsequence, the outside rule (cb_out
) is used for (base pairing) probabilities.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.- Bug:
Calling the
cb_out
callback is not implemented yet!
See also
vrna_gr_add_aux_exp_c(), vrna_gr_add_aux_exp_m(), vrna_gr_add_aux_exp_m1(), vrna_gr_add_aux_exp(), vrna_gr_add_aux_f(), vrna_gr_inside_exp_f, #vrna_gr_outside_exp_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_out – The auxiliary grammar callback for the outside (probability) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the partition function F-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_exp_c(vrna_fold_compound_t *fc, vrna_gr_inside_exp_f cb, vrna_gr_outside_exp_f cb_out, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/partfunc.h>
Add an auxiliary grammar rule for the C-decomposition (partition function version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the C-decomposition, i.e. the base pair decomposition stage.
While the inside rule (
cb
) computes the partition function for any subsequence, the outside rule (cb_out
) is used for (base pairing) probabilities.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.- Bug:
Calling the
cb_out
callback is not implemented yet!
See also
vrna_gr_add_aux_exp_f(), vrna_gr_add_aux_exp_m(), vrna_gr_add_aux_exp_m1(), vrna_gr_add_aux_exp(), vrna_gr_add_aux_c(), vrna_gr_inside_exp_f, #vrna_gr_outside_exp_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_out – The auxiliary grammar callback for the outside (probability) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the partition function C-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_exp_m(vrna_fold_compound_t *fc, vrna_gr_inside_exp_f cb, vrna_gr_outside_exp_f cb_out, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/partfunc.h>
Add an auxiliary grammar rule for the M-decomposition (partition function version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the M-decomposition, i.e. the multibranch loop decomposition stage.
While the inside rule (
cb
) computes the partition function for any subsequence, the outside rule (cb_out
) is used for (base pairing) probabilities.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.- Bug:
Calling the
cb_out
callback is not implemented yet!
See also
vrna_gr_add_aux_exp_f(), vrna_gr_add_aux_exp_c(), vrna_gr_add_aux_exp_m1(), vrna_gr_add_aux_exp(), vrna_gr_add_aux_m(), vrna_gr_inside_exp_f, #vrna_gr_outside_exp_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_out – The auxiliary grammar callback for the outside (probability) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the partition function M-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_exp_m1(vrna_fold_compound_t *fc, vrna_gr_inside_exp_f cb, vrna_gr_outside_exp_f cb_out, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/partfunc.h>
Add an auxiliary grammar rule for the M1-decomposition (partition function version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the M1-decomposition, i.e. the multibranch loop components with exactly one branch decomposition stage.
While the inside rule (
cb
) computes the partition function for any subsequence, the outside rule (cb_out
) is used for (base pairing) probabilities.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.- Bug:
Calling the
cb_out
callback is not implemented yet!
See also
vrna_gr_add_aux_exp_f(), vrna_gr_add_aux_exp_c(), vrna_gr_add_aux_exp_m(), vrna_gr_add_aux_exp(), vrna_gr_add_aux_m1(), vrna_gr_inside_exp_f, #vrna_gr_outside_exp_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_out – The auxiliary grammar callback for the outside (probability) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the partition function M1-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_exp_m2(vrna_fold_compound_t *fc, vrna_gr_inside_exp_f cb, vrna_gr_outside_exp_f cb_out, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/partfunc.h>
Add an auxiliary grammar rule for the M2-decomposition (partition function version)
This function binds callback functions for auxiliary grammar rules (inside and outside) in the M2-decomposition, i.e. the multibranch loop components with at least two branches.
While the inside rule (
cb
) computes the partition function for any subsequence, the outside rule (cb_out
) is used for (base pairing) probabilities.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.- Bug:
Calling the
cb_out
callback is not implemented yet!
See also
vrna_gr_add_aux_exp_f(), vrna_gr_add_aux_exp_c(), vrna_gr_add_aux_exp_m(), vrna_gr_add_aux_exp(), vrna_gr_add_aux_m1(), vrna_gr_inside_exp_f, #vrna_gr_outside_exp_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_out – The auxiliary grammar callback for the outside (probability) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for the partition function M2-decomposition stage, or 0 on error.
-
unsigned int vrna_gr_add_aux_exp(vrna_fold_compound_t *fc, vrna_gr_inside_exp_f cb, vrna_gr_outside_exp_f cb_out, void *data, vrna_auxdata_prepare_f data_prepare, vrna_auxdata_free_f data_release)
- #include <ViennaRNA/grammar/partfunc.h>
Add an auxiliary grammar rule (partition function version)
This function binds callback functions for auxiliary grammar rules (inside and outside) as additional, independent decomposition steps.
While the inside rule (
cb
) computes the partition function for any subsequence, the outside rule (cb_out
) is used for (base pairing) probabilities.Both callbacks will be provided with the
data
pointer that can be used to store whatever data is needed in the callback evaluations. Thedata_prepare
callback may be used to prepare thedata
just before the start of the recursions. If present, it will be called prior the actual decompositions automatically. You may use thedata_release
callback to properly free the memory ofdata
once it is not required anymore. Hence, it serves as a kind of destructor fordata
which will be called as soon as the grammar rules offc
are re-set to defaults or if thefc
is destroyed.- Bug:
Calling the
cb_out
callback is not implemented yet!
See also
vrna_gr_add_aux_exp_f(), vrna_gr_add_aux_exp_c(), vrna_gr_add_aux_exp_m(), vrna_gr_add_aux_exp_m1(), vrna_gr_add_aux(), vrna_gr_inside_exp_f, #vrna_gr_outside_exp_f, vrna_fold_compound_t, vrna_auxdata_prepare_f, vrna_auxdata_free_f
- Parameters:
fc – The fold compound that is to be extended by auxiliary grammar rules
cb – The auxiliary grammar callback for the inside step
cb_out – The auxiliary grammar callback for the outside (probability) step
data – A pointer to some data that will be passed through to the inside and outside callbacks
data_prepare – A callback to prepare
data
data_release – A callback to free-up memory occupied by
data
- Returns:
The current number of auxiliary grammar rules for partition function predictions, or 0 on error.
-
typedef struct vrna_gr_aux_s *vrna_gr_aux_t